Just looking for what is available on the net, i found lots an lots of nice implementations, however, they all were very complex, used a lot of javascript or everything was done with images/tables. I wanted a PHP/CSS solution without images, and in fact, it turned out very simple, and looks like this:
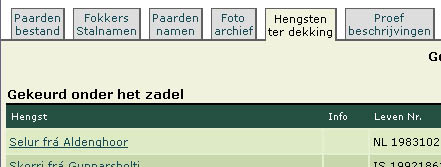
(no javascript and only 1 image used: a transparant dot (a gif file of 1x1 pixel, transparant)
Here is the class code:
class txtwebmenu {
var $objs; // all assigned objects
var $atxt = array();
var $aurl =array();
var $aopt =array();
var $nrobjs=0;
var $cursel="";
// additem - add a menu item,
// $item = the item ID
// $txt = default/normal image
// $url = jump-to url on select
function additem($item,$txt,$url="",$opt="") {
$this->atxt[$item] = $txt;
$this->aurl[$item] = $url;
$this->aopt[$item] = $opt; // options
}
// genmenu - the payoff: generate the whole thing
// $sel = item id of current selected item
function genmenu($sel="",$msize=2) {
if (strlen($sel)>0)
$this->cursel=$sel;
echo "<table border=0 cellpadding=0 cellspacing=0 width=100% bgcolor=white>";
echo "<tr><td height=3><img src='/ijs/gifs/transdot.gif' border=0></td></tr>";
echo "<tr><td>";
echo "<table border=0 cellpadding=$msize cellspacing=0>";
$ffirst=true;
reset($this->atxt);
while (list ($item, $txt) = each ($this->atxt)) {
echo ($ffirst) ? "<tr height=14><td class=twbspc><img src='/ijs/gifs/transdot.gif' border=0 width=6></td>" : "";
$target= (strchr($this->aopt[$item],"T") ? "target='_blank'" : "");
if ($this->cursel==$item) {
printf("<td class=twbmnuS align=center nowrap >");
echo "<a class='twbmnuS' href='".$this->aurl[$item]."' $target >$txt</a>";
}
else {
printf("<td class=twbmnu align=center nowrap>");
echo "<a class='twbmnu' href='".$this->aurl[$item]."' $target >$txt</a>";
}
echo "</td>";
echo "<td class=twbspc><img src='/ijs/gifs/transdot.gif' border=0 width=".($msize>1?"4":"2")."></td>";
$ffirst=false;
}
echo "<td class=twbspc width=100%><img src='/ijs/gifs/transdot.gif' border=0 width=1></td>";
echo "</tr>";
echo "</table>";
echo "</td></tr>";
echo "<tr><td bgcolor=#F2F1DD height=3><img src='/ijs/gifs/transdot.gif' border=0></td></tr>";
echo "</table>";
}
}
And here is the CSS. Acutally the whole "tab" look is done with borders in CSS:
TD.twbmnuS {font-family: verdana,arial,helvetica,sans-serif; font-size: 11px; font-weight: normal;
border-style:solid; border-color: #606060; background-color:#F2F1DD;
border-top-width: 1px;
border-left-width: 1px;
border-right-width: 2px;
border-bottom-width: 0px;
padding-left: 2px; padding-right:2px;}
TD.twbmnu {font-family: verdana,arial,helvetica,sans-serif; font-size: 11px; font-weight: normal;
border-style:solid; border-color: #606060; background-color:#DDDDDD;
border-top-width: 1px;
border-left-width: 1px;
border-right-width: 2px;
border-bottom-width: 2px;
padding-left: 2px; padding-right:2px; }
TD.twbspc {border-style:solid; border-color: #606060;
border-top-width: 0px; border-left-width: 0px; border-right-width: 0px; border-bottom-width: 2px; }
A.twbmnu:link {text-decoration: none; color: #164B56; xx: #567181;}
A.twbmnu:visited {text-decoration: none; color: #164B56; xx: #567181;}
A.twbmnu:Hover {text-decoration: underline; color: #000000; }
A.twbmnuS:link {text-decoration: none; color: #000000; }
A.twbmnuS:visited {text-decoration: none; color: #000000; }
A.twbmnuS:Hover {text-decoration: underline; color: #000000; }
And here is a sample how to use this class:
<?
$mo = new txtwebmenu; // a new tabmenu
$mo->additem('nr1','Item1',"item1.php?curmenu=nr1");
$mo->additem('nr2',"Item2","item2.php?curmenu=nr2");
?>
..html..
<? $mo->genmenu($curmenu); ?>
...html..
You could use separate php files for each tab-page, or use one "big" php file with all pages. With additem, you just supply the url of the page itself.
If you use separate php files, be sure to include the code above in every file (with an include)
The $curmenu var indicates which tab to highlight. Be sure to set it right, otherwise all tabls are grey
[ 25 comments ] ( 807 views ) | permalink | related link |





Changing from one editor to another... wow! An editor is a tool that i'm using so much, that changing from one to another feels like moving to another appartment.
On windows, I'm using homesite 3 for quite some years. Homesite 4 and 5 never attracted me: adding feature after feature to a product seldom makes it better. The new homesites were slower and less stable. So why change?
On unix, i'm using vi. But the reason for that is that its always available, and there are no alternatives, except for emacs, but that doesn't feel good. It has a steep learning curve, and i can't waste time learning an editor. On unix I stick with vi for a while.
But now, homesite 3 feels a bit outdated, and some issues are irritating.
So what are editor knock out criteria for me:
- stability. Losing a text file is not acceptable.
- lean and mean. Speed is essential
- correct tabs/indents. Most editors fail on this, create spaces instead of tabs etc.
- No silly mistakes with arrows and del keys. There are some simple rules that an editor needs to follow: backspace on the beginning of a line adds the line to the previous one, cursoring down may not change column position
- line numbers
- unlimited line length
- I like the homesite explorer-window on the left. It has become natural for me
- Multiple file editing, with one-click (tabbed) file changing
And a few nice to haves:
- ftp integration. Using an ftp-server as if you're working local (this is the reason I want to dismiss homesite3)
- overview/compression mode.
So I downloaded a few editors to see which one had it all. It turned out to be a dificult search:
Notepad++ - feel is good. However, no explorer toolwindow on the left.
Syn - No correct tab/indents. For the rest it seems ok
Jedit - ugly as hell (thanks to java), slow, no explorer window
MED - no correct tab/indents. Not lean and mean. Lot of useless stuff.
TexEdit - silly key mistakes. Does not feel natural. Bad indent/tab
Crimson - Feel is ok. No siilly mistakes. Tab/indent is a bit hidden, but the implementation is ok. It has a nice ftp feature, although not transparantly integrated it works ok. This is an editor that I will try for a while to see if it sticks.
[ add comment ] | permalink | related link |





Oracle installations are always a pain, and on a non-supported distro like gentoo it will probably be much worse. Lets see what we get.
The oracle CD (9.2) contains a script called runInstaller.
* Create a user oracle and group oracle to run this script in.
* run runInstaller from the cd as user oracle
The default gentoo fstab for cdroms does not allow running scripts from cd.
You will get a bash: permission denied error, even if the file is x-r.
(Oracle uses a shebang to sh instead of bash (#!/bin/sh) in their script, so i was first on the wrong track checking the sh link (/bin/sh -> /bin/bash) but that was fine)
the reason is the gentoo folks advise you to put the 'user' option on the cdrom mounts. But this also implies 'noexec'.
You need to add 'exec' to the /etc/fstab cdrom mount line options:
/dev/cdroms/cdrom0 /mnt/cdrom auto noauto,user,exec 0 0
* You need to emerge lib-compat. If not emerged, you'll get an error because oracle can't find libstdc++-libc6.1.1.so.2
The next the error is:
Unable to load native library: /tmp/OraInstall2005-01-02_07-02-08PM/jre/lib/i386 /libjava.so: symbol __libc_wait, version GLIBC_2.0 not defined in file libc.so.6 with link time reference.
See if I can fix this one in the next post.
[ 1 comment ] ( 338 views ) | permalink | related link |





after fiddling with mysql a bit, you normally want to use a real database. The limitations of mysql are numerous: no views, no triggers, no stored procedures and so on. For very simple applications its ok, but sql is more than just a select statement. Therefore someday you move over to oracle. Oracle software normally sucks, but their sql server rocks. Its very stable, scalable, unix oriented stuff.
Some do's and don'ts:
- never run oracle on windows. Its not stable (even less stable than windows itself). It is just not a good combination. You need to reboot it all the time (at least daily) due to memory leaks. Prepare for the worst if you're following this path.
- Never use other oracle software than their database server. Oracle forms? a joke. Oracle designer? sucks. Oracle installations: Always a pain. I have *NEVER* seen an oracle installation procedure without severe errors. (and that means over 7 years of oracle installtion history)
- Oracle databases are memory hungry. Start with 1Gb, then go up.
- After install, immediately tune up the server parameters. The defaults are not usable. Make your shared pool at least 500M for a 1Gb server, use large dbblocks (16k at least) If you don't do this, only 2 users can log in
[ 1 comment ] ( 26 views ) | permalink | related link |





Assuming you can connect locally to mysql, you'd expect to be able to connect remote...not! This is not as easy as it seems.
First make sure you CAN access the mysql server from a remote machine. By default, the my.cnf file contains a statement:
bind-address=127.0.0.1
that is in your way. This only allows connections from the localhost. Put a # in front of it.
After this, still no luck, because the mysql privilege system is not your default user/password pair, but mysql for some reason also needs to know where you're calling from. This is due to the unusual non-standard structure of their user table: it contains host/user/password.
So add your remote host to the table by issuing:
grant all on *.* to user@remotehost identified by 'password'
or, if you want to allow access from all hosts, use a % like:
grant all on *.* to 'user'@'%' identified by 'password'
note: put quotes around username and host if neccesary. Beware not to quote the complete thing (like 'user@host') because mysql thinks you mean user "user@host" on the local machine.
Because mysql caches privileges, you need to flush them so enter:
flush privileges
after this you should be able to login
to see if its ok, do
use mysql
select * from user;
That's it. Sounds easy, but as the mysql documentation index/search sucks bigtime it can take you hours to find this out. (for instance, searching on the 1130 error number puts you on the wrong trail by giving information about blocked-hosts that has nothing to do with the real problem)
[ 2 comments ] ( 227 views ) | permalink | related link |





Back Next